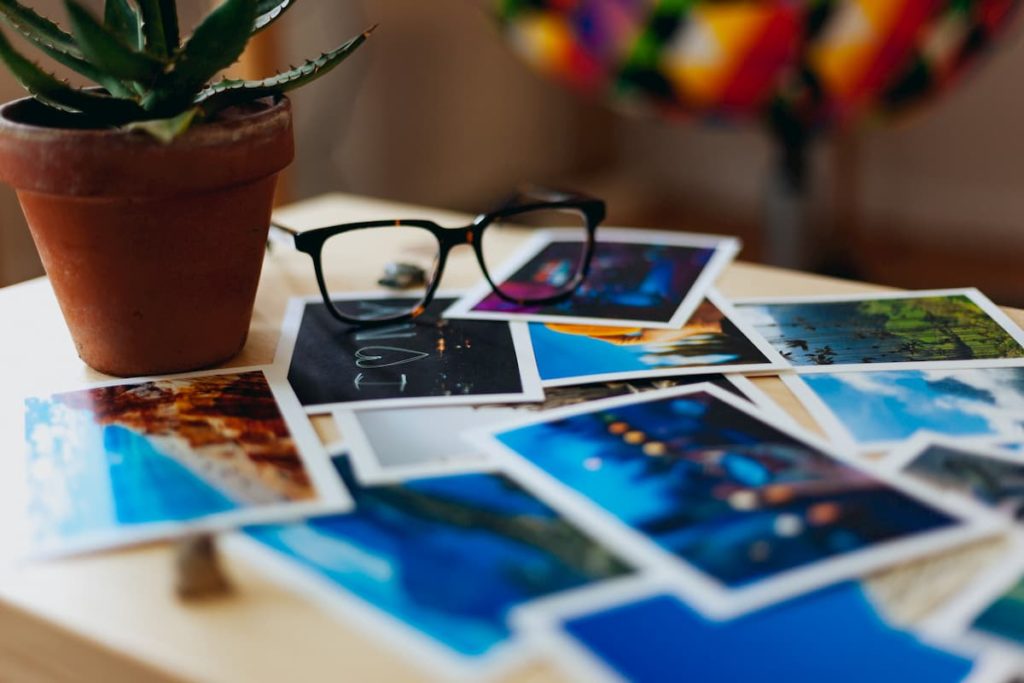
One of the greatest things about WordPress, a CMS that runs about 35% of the internet, is the flexibility of it. You can do just about anything with WordPress or the plethora of unending plugins available for download. WordPress’ back end is so robust that there are plenty of features built in that you don’t necessarily need to download a plugin to get what you need. With a little bit of knowledge, and some fiddling in the back end (make sure you do a backup prior to any work!), you can achieve impressive results.
One thing WordPress has, by default, is a variety of image sizes that you can use on your blog, pages, or in your website’s design. But WordPress’ default image sizes aren’t exhaustive, and perhaps you’re looking for a way to use other sizes. We’ll explain all about WordPress’ image sizes and how to register your own image sizes within WordPress.
Why image sizes?
It’s important to note that for any graphic uploaded to WordPress, WordPress will automatically create a variety of sizes of that image, as well as compress those additional image sizes. WordPress does this to help keep down page load speed.
Here’s an example:
If you upload a 20 mb image from your iPhone that’s 6000×4000 pixels in size, when you place that on your page, regardless of the size you determine it to be viewed at (let’s say you tell Gutenberg to display it at 50% instead), your visitors will still need to download the whole 20 mb of that file into their cache in order to see the photo. This is essentially what happens when you tell Gutenberg to use the “full” image in a block.
WordPress automatically creates multiple copies of your image at various sizes when you upload your “full” image. This way, you will have a few sizes to choose from when using the image on your WordPress site. The caveat here is that since WordPress is creating multiple files, you can eat up web space very quickly if you have a lot of image sizes registered.
Still, if you have a spot where you need only square images, you may want to think about registering a square WordPress image size so you can have WordPress do the heavy lifting for you.
A note about compression
WordPress, by default, compresses the images it makes with a quality of 80%. If you need this to be a lower quality (saving space), or higher quality, you can change it in your functions.php:
add_filter('jpeg_quality', function($arg){return 100;});
Note the “return” number is the quality, with 100 being the highest quality, and 1 being the lowest.
What image sizes are registered by default?
WordPress, out of the box, has a few default image sizes that are usually just fine for most implementations. Here are those sizes:
Thumbnail: 150x150px square
Medium: Max 300px width or height (whichever hits it first)
Large: Max 1024px width or height (whichever hits it first)
Full size: The original image you uploaded
These are not the same as the image sizes in the Gutenberg blocks, as you can select a “large” size image and then reduce it down further by percentage, if you want. Still, if you select “large” and reduce it by 50% in the block, it’s still going to load the full “large” size image.
How can I register a new image size?
You may find that you need a new image size for somewhere on your site. For example, I wanted an image that served only for my RSS feed, with a max width of 500px. I need to determine what kind of size and crop I want on the automatically created image, so understanding how the parameters of the code work is important.
add_image_size( string $name, int $width, int $height, bool|array $crop = false )
Let’s make this a little easier to understand.
add_image_size( ‘the-name-of-the-image-size’, max width in pixels, max height in pixels, crop? (true, false, or array) );
In my instance, all I cared about was having the max width be 500 px. So I registered in my functions.php:
add_image_size( 'rss-image', 500 );
If I wanted it to be square, I’d register:
add_image_size( 'rss-image', 500, 500 );
If I want WordPress to crop my image, I will write:
add_image_size( 'rss-image', 500, 500, true );
If I do not want WordPress to crop my image (and instead just scale it), I will write:
add_image_size( 'rss-image', 500, 500, false );
If I want WordPress to crop my image, but I want to tell it where to crop, let’s say I want it in the upper right, I’d write:
add_image_size( 'rss-image', 500, 500, array( 'right', 'top') );
Your first value for cropping is the x axis, and the second value is the y axis. X accepts “left”, “center”, or “right” for a value, while Y accepts “top”, “center”, and “bottom” for a value. If no value is assigned, WordPress will assume “center” for both x and y values.
How to use your new image sizes
If you’re just using the image size internally, you can use your new image size, in my instance, “rss-image”, as you would use “large”, “full”, or any of the others. For example:
the_post_thumbnail('rss-image');
Would produce the new image size at 500 px max width for featured images.
If you want to be able to see the size in the media center, there are a couple of extra steps.
You’ve registered your new image size with WordPress, but you’ll also need to register it with the media center. Here’s how:
add_filter( 'image_size_names_choose', 'new_image_sizes' );
function new_image_sizes( $sizes ) {
return array_merge( $sizes, array(
'rss-image' => __( 'RSS Image 500px max width' ),
) );
}
Add this to functions.php in your theme. This will add our new image into the media center, and make it selectable in Gutenberg blocks as an option. If you have more than one image size to add, you can add each without repeating the filter.
Where are all my images?
You may notice that if you just registered a new image size, not all of your images will have been created with that new image size. In order to do that, you will need to force WordPress to regenerate your image files. There’s a handy plugin that can do this easy called Regenerate Thumbnails. Yes, this can be completed faster using WP-CLI and can be done without the use of a plugin, however the plugin is so simple and easy to use, and you can uninstall it when you’re done.
How to remove unused sizes
If you’re looking to conserve space on a hosting environment, you may want to remove image sizes that aren’t used. Sometimes themes can register image sizes and cause unnecessary bloat on the server. Here’s how you can remove an image size from occurring:
function remove_image_sizes() {
remove_image_size( 'rss-image');
}
add_action('init', 'remove_image_sizes');
That’s it! Add it to your functions.php and the image size will cease to be there… Better yet, WordPress will stop creating images in that size.
How are you using your custom image sizes? Let us know in the comments below!
Nice list of functions, especially the last one for removing unused images. I’m sure readers will find that one especially useful for improving performance.